0. 서론
Typescript 맛보기 1편으로 Typescript를 Javascript로 compile 하는 방법을 알아보자.
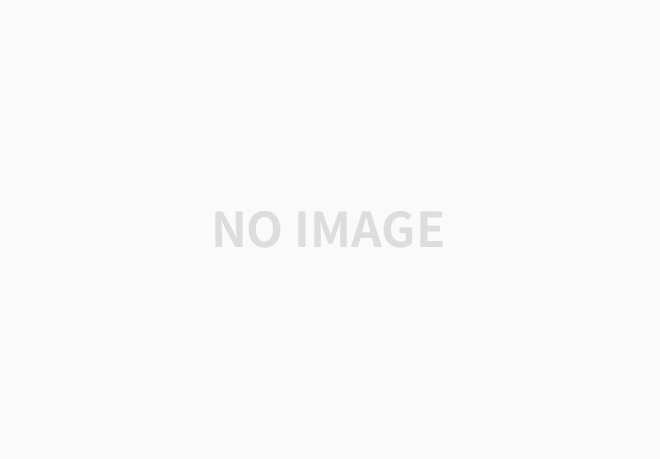
1. 간단히 따라해 보기
Typescript는 직접 실행 시킬 수 없기 때문에(불가능 한 것은 아니다) Javascript로 compile 시켜야 코드를 실행시킬 수 있다. 간단히 따라해보면서 Typescript를 Javascript로 Compile 하는 방법을 맛보자.
실습환경은 nodejs만 다룰 줄 알면 된다.
a. typescript 설치&설정
typescript를 devDependencies로 설치한다.
$ npm i -D typescript // typescript를 devDependencies에 설치
다음을 입력하면 프로젝트 디렉토리에 tsconfig.json이 생성된다.
$ tsc --init
tsconfig.json를 열어보면 compilerOptions의 여러 props과 comments를 볼 수 있다. 이것에 대한 더 자세한 설명은 여기(https://www.typescriptlang.org/docs/handbook/tsconfig-json.html)를 참고하자.
tsconfig.json 혹은 compile에 대한 옵션을 담고 있는 .json 파일이 있다면, 그 파일을 참조해 tsc 명령어로 컴파일 할 수 있게 된다. 따라서 적절히 compile하기 위해, 아래와 같이 tsconfig.json을 수정하자.
props 중 한 가지만 간단히 설명하자면, outFile은 컴파일시킨 목적지를 일컫는다. 나중에 이 것이 적용됐나 확인해 볼 것이다.
// tsconfig.json
{
"compilerOptions": {
"module": "system",
"target": "es6",
"noImplicitAny": true,
"removeComments": true,
"preserveConstEnums": true,
"outFile": "built/local/tsc.js",
"sourceMap": true
},
"include": [
"src/**/*"
],
"exclude": [
"node_modules",
"**/*.spec.ts"
]
}
b. typescript 코딩
src/index.ts 에 아래와 같이 typescript 코드를 작성해보자. class내의 private 멤버변수, interface 등은 Javascript만 사용했다면 낯설것이다.
주의할 점은 class의 getter는 compilerOptions.target이 es6 이상일 때만 컴파일 된다.
// src/index.ts
class Student {
private _fullName: string;
constructor(person: Person) {
this._fullName = person.firstName + " " + person.middleName + " " + person.lastName;
}
get fullName(): string {
return this._fullName;
}
}
interface Person {
firstName: string;
middleName?: string;
lastName: string;
}
let user = new Student({firstName: "Jane", lastName: "Jung"});
console.log(user.fullName);
c. 컴파일
아래와 같이 "tsc"만 입력하면 알아서 tsconfig.json의 설정을 읽어들여, compilerOptions.outFile에 지정한 "built/local/tsc.js"에 .js 파일이 컴파일 될 것이다. 어떻게 설정파일을 읽고, 확장가능한가에 대해 궁금하면, https://www.typescriptlang.org/docs/handbook/tsconfig-json.html#configuration-inheritance-with-extends 를 읽어보면 된다.
$ tsc
d. 컴파일된 소스
src/index.ts를 컴파일한 소스를 살펴보자. s
// built/local/tsc.js
class Student {
constructor(person) {
this._fullName = person.firstName + " " + person.middleName + " " + person.lastName;
}
get fullName() {
return this._fullName;
}
}
let user = new Student({ firstName: "Jane", lastName: "Jung" });
console.log(user.fullName);
//# sourceMappingURL=tsc.js.map
실행 시켜면 당연히? 잘 동작한다.
$ node built/local/tsc.js
>> Jane undefined Jung
3. 결론
Typescript에 대해 정말 간단히 맛보기를 해봤다. 다음 번에는 Typescript기반 Koa(https://koajs.com/) 백엔드 개발 환경을 구축햐보자.
'Programming language > Typescript' 카테고리의 다른 글
TypeScript, any와 unknown 타입 비교 (0) | 2020.11.29 |
---|---|
TypeScript class를 JavaScript ES5, ES6 code로 변환하면서 (0) | 2020.09.03 |
TypeScript 맛보기 4 - ESLint 사용하기 (0) | 2019.11.03 |
Typescript 맛보기 3 - VS Studio Code에서 Prettier 적용하기 (0) | 2019.11.02 |
Typescript 맛보기 2 - 백엔드 Koa 개발환경 설정 (0) | 2019.11.01 |